GSoC Status Report: Code Completion features
Context: I'm currently working on getting Clang integration in KDevelop into usable shape as part of this year's Google Summer of Code. See the initial blog post for my road map.
While we had basic support for code completion provided by Clang from the beginning (thanks to David Stevens for the initial) work, it still didn't really feel that useful in most cases. In the last two weeks I've spent my time streamlining the code completion features provided by Clang.
This blog post is going to be full of screenshots showing the various features we've been working on lately.
Task recap: Code completion
-
Code completion: Implement “virtual override completion”. Also the automatic replacement of
.
to->
and vice-versa in case of pointer/non-pointer types is still missing. -
“Implement function” feature: If a function is only declared but not defined, we offer a "implement function" helper item in the code completion. This is currently not yet ported to clangcpp.
-
"Switch to Definition/Declaration” feature: If the cursor is at some declaration in a header file, KDevelop offers a shortcut to automatically switch to its definition in the source file (opening the corresponding file in the active view). This is not yet possible in clangcpp.
-
Show viable expressions for current context: When inside a function call or constructor call, show viable expressions which fit the signature of the current argument. Example:
int i = 1; char* str = “c”; strlen(
– this should show variablestr
in the completion popup as best match. -
Include completion: Oldcpp offers completion hints when attempting to
#include
some file, port this to clangcpp.
Achievements
Virtual override completion
Simple case
When in a class context, we can now show completion items for methods that are declared virtual
in the base class.
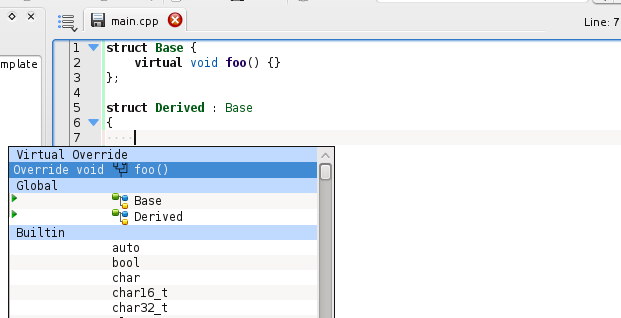
By pressing Enter now, KDevelop automatically inserts the following code at the current cursor position:
virtual void foo()
Oh, no! Templates!
We've spent a bit of work to make this feature work with templated base classes, too. Have a look at this:
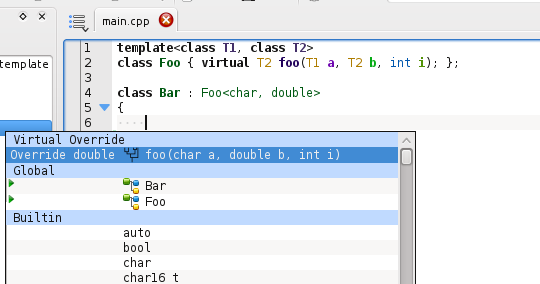
Nice, right?
Implement function helper
When encountering an undefined method which is reachable from within the current context, KDevelop offers to implement those via a tooltip
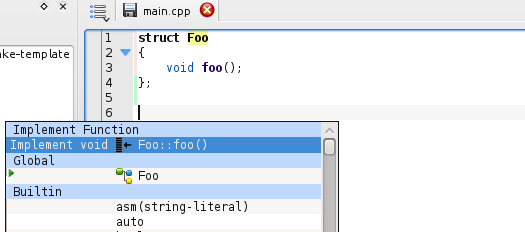
By pressing Enter now, KDevelop automatically inserts the following code at the current cursor position:
void Foo::foo()
{
}
This works for all types of functions, be it class member functions or free member functions and/or functions in namespaces. Since this is mostly the same code path as the "virtual override helper" feature, this plays nicely with templated functions, too.
"Switch to Definition/Declaration” feature
Sorry, no pictures here, but be assured: It works!
Pressing Ctrl+, ("Jump to Definition") while having the cursor on some declaration will bring you to the definition. Consecutively, pressing Ctrl+. ("Jump to Declaration") on some definition will bring you to the declaration of that definition.
Show viable expressions for current context
Best matches
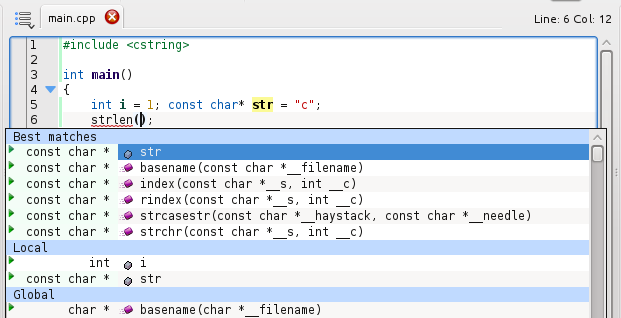
This is some of the features we actually get for free when using Clang. We get the completion results by invoking clang_codeCompleteAt(...)
on the current translation unit and iterating through the results libclang is offering us. Clang gives highly useful completion results, the LLVM team did an amazing job here.
Another example: Enum-case completion
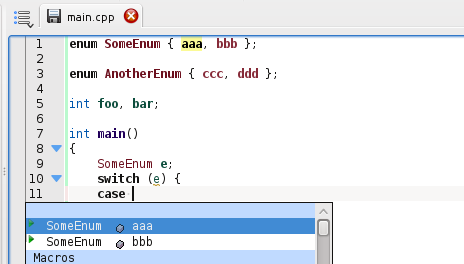
You can play around with Clang's code completion ability from the command-line. Consider the following code in some file test.cpp:
enum SomeEnum { aaa, bbb };
int main()
{
SomeEnum e;
switch (e) {
case
// ^- cursor here
}
}
Now do clang++ -cc1 -x c++ -fsyntax-only -code-completion-at -:7:9 - < test.cpp
and you'll get:
COMPLETION: aaa : [#SomeEnum#]aaa
COMPLETION: bbb : [#SomeEnum#]bbb
Awesome, right?
Issues: Too many code completion result from Clang
One thing I've found a bit annoying about the results we're getting is that Clang also proposes to explicitly call the constructors/destructors or assignment operators in some cases. Or in other words: It proposes too many items
Consider the following code snippet:
struct S
{
void foo();
};
int main()
{
S s;
s.
//^- cursor here
}
Now doing clang++ -cc1 -x c++ -fsyntax-only -code-completion-at -:8:7 - < test.cc
results in:
COMPLETION: foo : [#void#]foo()
COMPLETION: operator= : [#S &#]operator=(<#const S &#>)
COMPLETION: S : S::
COMPLETION: ~S : [#void#]~S()
Using one of the last three completion results would encourage Clang to generate code such as s.S
, s.~S
or s.operator=
. While these constructs point to valid symbols, this is likely undesired.
Solution: We filter out everything that looks like a constructor, destructor or operator declaration by hand.
So, in fact, what we end up showing the user inside KDevelop is:
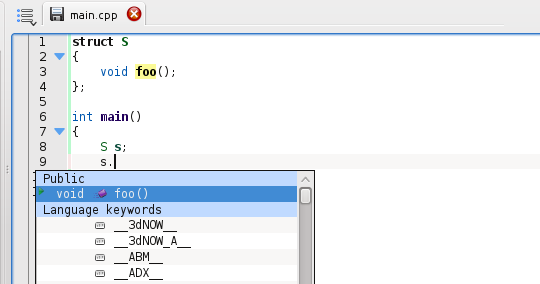
Just what you'd expect.
Wrap-Up
Code completion features are mostly done (at least from the point-of-view of what Clang can give us here).
Still, there other interesting completion helpers that could^Wshould be ported over from oldcpp to kdev-clang, such as Olivier's lookahead-completion feature (which I find quite handy). This is not yet done.
I'm writing up yet another blog post which is going to highlight some of the other bits and pieces I've been busy with during the last weeks.
Thanks!